Button Buttons
Options
Use any of the available button style types to quickly create a styled button. Just modify the bsStyle
prop.
Button spacing
Because React doesn't output newlines between elements, buttons on the same line are displayed flush against each other. To preserve the spacing between multiple inline buttons, wrap your button group in <ButtonToolbar />
.
Sizes
Fancy larger or smaller buttons? Add bsSize="large"
, bsSize="small"
, or bsSize="xsmall"
for additional sizes.
Create block level buttons—those that span the full width of a parent— by adding the block
prop.
Active state
To set a buttons active state simply set the components active
prop.
Button tags
The DOM element tag is choosen automatically for you based on the props you supply. Passing a href
will result in the button using a <a />
element otherwise a <button />
element will be used.
Disabled state
Make buttons look unclickable by fading them back 50%. To do this add the disabled
attribute to buttons.
Cross-Browser compatibility
Because <Button />
will render an <a>
tag in certain situations. Since anchor tags can't be disabled
, the behavior is emulated as best it can be. Specifically, pointer-events: none;
style is added to the anchor to prevent focusing, which is only supported in newer browser versions.
Button loading state
When activating an asynchronous action from a button it is a good UX pattern to give the user feedback as to the loading state, this can easily be done by updating your <Button />
’s props from a state change like below.
Props
Name | Type | Default | Description |
---|---|---|---|
active | boolean | false | |
block | boolean | false | |
bsClass | string | 'btn' | Base CSS class and prefix for the component. Generally one should only change |
bsSize | one of: "lg" , "large" , "sm" , "small" , "xs" , "xsmall" | Component size variations. | |
bsStyle | one of: "success" , "warning" , "danger" , "info" , "default" , "primary" , "link" | 'default' | Component visual or contextual style variants. |
componentClass | elementType | You can use a custom element type for this component. | |
disabled | boolean | false | |
href | string | ||
onClick | function | ||
type | one of: 'button' , 'reset' , 'submit' | 'button' | Defines HTML button type attribute |
ButtonGroup, ButtonToolbar, ToggleButtonGroup, ToggleButton Button groups
Group a series of buttons together on a single line with the button group.
Basic example
Wrap a series of <Button />
s in a <ButtonGroup />
.
Button toolbar
Combine sets of <ButtonGroup />
s into a <ButtonToolbar />
for more complex components.
Sizing
Instead of applying button sizing props to every button in a group, just add bsSize
prop to the <ButtonGroup />
.
Nesting
You can place other button types within the <ButtonGroup />
like <DropdownButton />
s.
Checkbox / Radio
For checkboxes or radio buttons styled as Bootstrap buttons, use the <ToggleButtonGroup>
and <ToggleButton>
components. The group behaves as a form component, where the value is an array of the selected eventKey
s for checkbox groups or the selected eventKey
for radio groups.
Uncontrolled
Controlled
Vertical variation
Make a set of buttons appear vertically stacked rather than horizontally. Split button dropdowns are not supported here.
Just add vertical
to the <ButtonGroup />
.
Moreover, you can have buttons be block level elements so they take the full width of their container, just add block
to the <ButtonGroup />
, in addition to the vertical
you just added.
Justified button groups
Make a group of buttons stretch at equal sizes to span the entire width of its parent. Also works with button dropdowns within the button group.
Style issues
There are some issues and workarounds required when using this property, please see bootstrap’s button group docs for more specifics.
Just add justified
to the <ButtonGroup />
.
Props
ButtonGroup
Name | Type | Default | Description |
---|---|---|---|
block | boolean | false | Display block buttons; only useful when used with the "vertical" prop. |
bsClass | string | 'btn-group' | Base CSS class and prefix for the component. Generally one should only change |
justified | boolean | false | |
vertical | boolean | false |
ButtonToolbar
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'btn-toolbar' | Base CSS class and prefix for the component. Generally one should only change |
ToggleButtonGroup
Name | Type | Default | Description |
---|---|---|---|
name | string | An HTML Required if | |
onChange | function | controls values Callback fired when a button is pressed, depending on whether the | |
type required | one of: 'checkbox' , 'radio' | 'radio' | The input |
value | any | controlled by: onChange , initial prop: defaultValue The value, or array of values, of the active (pressed) buttons |
ToggleButton
Name | Type | Default | Description |
---|---|---|---|
checked | boolean | The checked state of the input, managed by ` | |
disabled | boolean | The disabled state of both the label and input | |
name | string | The HTML input name, used to group like checkboxes or radio buttons together semantically | |
onChange | function | [onChange description] | |
type | [type] | The | |
value required | any | The value of the input, and unique identifier in the ToggleButtonGroup |
DropdownButton, SplitButton, Dropdown Dropdowns
Single button dropdowns
Create a dropdown button with the <DropdownButton />
component.
Split button dropdowns
Similarly, create split button dropdowns with the <SplitButton />
component.
Sizing
Dropdowns work with buttons of all sizes.
No caret variation
Remove the caret using the noCaret
prop.
Dropup variation
Trigger dropdown menus that site above the button by adding the dropup
prop.
Dropdown right variation
Trigger dropdown menus that align to the right of the button using the pullRight
prop.
Dropdown Customization
If the default handling of the dropdown menu and toggle components aren't to your liking, you can customize them, by using the more basic Dropdown
Component to explicitly specify the Toggle and Menu components
Additional Import Options
As a convenience Toggle and Menu components available as static properties on the Dropdown component. However, you can also import them directly, from the /lib
directory like: require("react-bootstrap/lib/DropdownToggle")
.
Custom Dropdown Components
For those that want to customize everything, you can forgo the included Toggle and Menu components, and create your own. In order to tell the Dropdown component what role your custom components play, add a special prop bsRole
to your menu or toggle components. The Dropdown expects at least one component with bsRole="toggle"
and exactly one with bsRole="menu"
. Custom toggle and menu components must be able to accept refs.
Props
DropdownButton
Name | Type | Default | Description |
---|---|---|---|
bsSize | string | Component size variations. | |
bsStyle | string | Component visual or contextual style variants. | |
componentClass | elementType | ButtonGroup | You can use a custom element type for this component. |
defaultOpen | boolean | ||
disabled | boolean | Whether or not component is disabled. | |
dropup | boolean | The menu will open above the dropdown button, instead of below it. | |
id required | string|number | An html id attribute, necessary for assistive technologies, such as screen readers. | |
noCaret | boolean | ||
onSelect | function | A callback fired when a menu item is selected.
| |
onToggle | function | controls open A callback fired when the Dropdown wishes to change visibility. Called with the requested
| |
open | boolean | controlled by: onToggle , initial prop: defaultOpen Whether or not the Dropdown is visible. | |
pullRight | boolean | Align the menu to the right side of the Dropdown toggle | |
role | string | If | |
rootCloseEvent | one of: 'click' , 'mousedown' | Which event when fired outside the component will cause it to be closed | |
title required | node |
SplitButton
Name | Type | Default | Description |
---|---|---|---|
bsSize | string | Component size variations. | |
bsStyle | string | Component visual or contextual style variants. | |
componentClass | elementType | ButtonGroup | You can use a custom element type for this component. |
defaultOpen | boolean | ||
disabled | boolean | Whether or not component is disabled. | |
dropup | boolean | The menu will open above the dropdown button, instead of below it. | |
href | string | ||
id required | string|number | An html id attribute, necessary for assistive technologies, such as screen readers. | |
onClick | function | ||
onSelect | function | A callback fired when a menu item is selected.
| |
onToggle | function | controls open A callback fired when the Dropdown wishes to change visibility. Called with the requested
| |
open | boolean | controlled by: onToggle , initial prop: defaultOpen Whether or not the Dropdown is visible. | |
pullRight | boolean | Align the menu to the right side of the Dropdown toggle | |
role | string | If | |
rootCloseEvent | one of: 'click' , 'mousedown' | Which event when fired outside the component will cause it to be closed | |
title required | node | The content of the split button. | |
toggleLabel | string | Accessible label for the toggle; the value of |
Dropdown
Name | Type | Default | Description |
---|---|---|---|
children | node | The children of a Dropdown may be a | |
componentClass | elementType | ButtonGroup | You can use a custom element type for this component. |
defaultOpen | boolean | ||
disabled | boolean | Whether or not component is disabled. | |
dropup | boolean | The menu will open above the dropdown button, instead of below it. | |
id required | string|number | An html id attribute, necessary for assistive technologies, such as screen readers. | |
onSelect | function | A callback fired when a menu item is selected.
| |
onToggle | function | controls open A callback fired when the Dropdown wishes to change visibility. Called with the requested
| |
open | boolean | controlled by: onToggle , initial prop: defaultOpen Whether or not the Dropdown is visible. | |
pullRight | boolean | Align the menu to the right side of the Dropdown toggle | |
role | string | If | |
rootCloseEvent | one of: 'click' , 'mousedown' | Which event when fired outside the component will cause it to be closed |
MenuItem Menu items
This component represents a menu item in a dropdown.
It supports the basic anchor properties href
, target
, title
.
It also supports different properties of the normal Bootstrap MenuItem.
header
: To add a header label to sectionsdivider
: Adds an horizontal divider between sectionsdisabled
: shows the item as disabled, and preventsonSelect
from firingeventKey
: passed to the callbackonSelect
: a callback that is called when the user clicks the item.
The callback is called with the following arguments: event
and eventKey
Props
Name | Type | Default | Description |
---|---|---|---|
active | boolean | Highlight the menu item as active. | |
bsClass | string | 'dropdown' | Base CSS class and prefix for the component. Generally one should only change |
disabled | boolean | false | Disable the menu item, making it unselectable. |
divider | all | false | Styles the menu item as a horizontal rule, providing visual separation between groups of menu items. |
eventKey | any | Value passed to the | |
header | boolean | false | Styles the menu item as a header label, useful for describing a group of menu items. |
href | string | HTML | |
onClick | function | Callback fired when the menu item is clicked. | |
onSelect | function | Callback fired when the menu item is selected.
|
Overlays
React-Bootstrap offers a number of accessible overlay components built using react-overlays.
Modal Modals
Static Markup
A modal dialog component
Basic example
A modal with header, body, and set of actions in the footer. Use <Modal/>
in combination with other components to show or hide your Modal. The <Modal/>
Component comes with a few convenient "sub components": <Modal.Header/>
, <Modal.Title/>
, <Modal.Body/>
, and <Modal.Footer/>
, which you can use to build the Modal content.
Click to get the full Modal experience!
Additional Import Options
The Modal Header, Title, Body, and Footer components are available as static properties the <Modal/>
component, but you can also, import them directly from the /lib
directory like: require("react-bootstrap/lib/ModalHeader")
.
Contained Modal
You will need to add the following css to your project and ensure that your container has the modal-container
class.
.modal-container {
position: relative;
}
.modal-container .modal, .modal-container .modal-backdrop {
position: absolute;
}
Sizing modals using standard Bootstrap props
You can specify a bootstrap large or small modal by using the "bsSize" prop.
Sizing modals using custom CSS
You can apply custom css to the modal dialog div using the "dialogClassName" prop. Example is using a custom css class with width set to 90%.
Props
Modal
Name | Type | Default | Description |
---|---|---|---|
animation | boolean | true | Open and close the Modal with a slide and fade animation. |
autoFocus | boolean | When | |
backdrop | one of: 'static' , true , false | Include a backdrop component. Specify 'static' for a backdrop that doesn't trigger an "onHide" when clicked. | |
backdropClassName | string | Add an optional extra class name to .modal-backdrop It could end up looking like class="modal-backdrop foo-modal-backdrop in". | |
bsClass | string | 'modal' | Base CSS class and prefix for the component. Generally one should only change |
bsSize | one of: "lg" , "large" , "sm" , "small" | Component size variations. | |
dialogClassName | string | A css class to apply to the Modal dialog DOM node. | |
dialogComponentClass | elementType | ModalDialog | A Component type that provides the modal content Markup. This is a useful prop when you want to use your own styles and markup to create a custom modal component. |
enforceFocus | boolean | When | |
keyboard | boolean | Close the modal when escape key is pressed | |
onEnter | function | Callback fired before the Modal transitions in | |
onEntered | function | Callback fired after the Modal finishes transitioning in | |
onEntering | function | Callback fired as the Modal begins to transition in | |
onExit | function | Callback fired right before the Modal transitions out | |
onExited | function | Callback fired after the Modal finishes transitioning out | |
onExiting | function | Callback fired as the Modal begins to transition out | |
onHide | function | A callback fired when the header closeButton or non-static backdrop is clicked. Required if either are specified. | |
restoreFocus | boolean | When | |
show | boolean | When |
Modal.Header
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'modal-header' | Base CSS class and prefix for the component. Generally one should only change |
closeButton | boolean | false | Specify whether the Component should contain a close button |
closeLabel | string | 'Close' | Provides an accessible label for the close button. It is used for Assistive Technology when the label text is not readable. |
onHide | function | A Callback fired when the close button is clicked. If used directly inside
a Modal component, the onHide will automatically be propagated up to the
parent Modal |
Modal.Title
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'modal-title' | Base CSS class and prefix for the component. Generally one should only change |
componentClass | elementType | 'h4' | You can use a custom element type for this component. |
Modal.Body
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'modal-body' | Base CSS class and prefix for the component. Generally one should only change |
componentClass | elementType | 'div' | You can use a custom element type for this component. |
Modal.Footer
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'modal-footer' | Base CSS class and prefix for the component. Generally one should only change |
componentClass | elementType | 'div' | You can use a custom element type for this component. |
Tooltip Tooltips
Tooltip component for a more stylish alternative to that anchor tag title
attribute.
With OverlayTrigger
Attach and position tooltips with OverlayTrigger
.
In text copy
Positioned tooltip in text copy.
Tight pants next level keffiyeh you probably haven't heard of them. Photo booth beard raw denim letterpress vegan messenger bag stumptown. Farm-to-table seitan, mcsweeney's fixie sustainable quinoa 8-bit american apparel have aterry richardson vinyl chambray. Beard stumptown, cardigans banh mi lomo thundercats. Tofu biodiesel williamsburg marfa, four loko mcsweeney's cleanse vegan chambray. A really ironic artisan whatever keytar, scenester farm-to-table banksy Austin twitter handle freegan cred raw denim single-origin coffee viral.
Props
OverlayTrigger
Name | Type | Default | Description |
---|---|---|---|
animation | boolean | elementType | Fade | Use animation |
defaultOverlayShown | boolean | false | The initial visibility state of the Overlay. For more nuanced visibility control, consider using the Overlay component directly. |
delay | number | A millisecond delay amount to show and hide the Overlay once triggered | |
delayHide | number | A millisecond delay amount before hiding the Overlay once triggered. | |
delayShow | number | A millisecond delay amount before showing the Overlay once triggered. | |
onEnter | function | Callback fired before the Overlay transitions in | |
onEntered | function | Callback fired after the Overlay finishes transitioning in | |
onEntering | function | Callback fired as the Overlay begins to transition in | |
onExit | function | Callback fired right before the Overlay transitions out | |
onExited | function | Callback fired after the Overlay finishes transitioning out | |
onExiting | function | Callback fired as the Overlay begins to transition out | |
overlay required | node | An element or text to overlay next to the target. | |
placement | one of: 'top' , 'right' , 'bottom' , 'left' | 'right' | Sets the direction of the Overlay. |
rootClose | boolean | false | Specify whether the overlay should trigger onHide when the user clicks outside the overlay |
trigger | triggerType | array<triggerType> | ['hover', 'focus'] | Specify which action or actions trigger Overlay visibility |
Tooltip
Name | Type | Default | Description |
---|---|---|---|
arrowOffsetLeft | number | string | The "left" position value for the Tooltip arrow. | |
arrowOffsetTop | number | string | The "top" position value for the Tooltip arrow. | |
bsClass | string | 'tooltip' | Base CSS class and prefix for the component. Generally one should only change |
id required | string|number | An html id attribute, necessary for accessibility | |
placement | one of: 'top' , 'right' , 'bottom' , 'left' | 'right' | Sets the direction the Tooltip is positioned towards. |
positionLeft | number | string | The "left" position value for the Tooltip. | |
positionTop | number | string | The "top" position value for the Tooltip. |
Popover Popovers
The Popover, offers a more robust alternative to the Tooltip for displaying overlays of content.
Popover right
With OverlayTrigger
The Popover component, like the Tooltip can be used with an OverlayTrigger
Component, and positioned around it.
Trigger behaviors
It's inadvisable to use "hover"
or "focus"
triggers for popovers, because they have poor accessibility from keyboard and on mobile devices.
Popover component in container
Specify container
to control the DOM element to which to append the overlay. This element must be a positioned element to allow correctly positioning the overlay.
Positioned popover components in scrolling container
Props
Name | Type | Default | Description |
---|---|---|---|
arrowOffsetLeft | number | string | The "left" position value for the Popover arrow. | |
arrowOffsetTop | number | string | The "top" position value for the Popover arrow. | |
bsClass | string | 'popover' | Base CSS class and prefix for the component. Generally one should only change |
id required | string | An html id attribute, necessary for accessibility | |
placement | one of: 'top' , 'right' , 'bottom' , 'left' | 'right' | Sets the direction the Popover is positioned towards. |
positionLeft | number | string | The "left" position value for the Popover. | |
positionTop | number | string | The "top" position value for the Popover. | |
title | node | Title content |
Overlay Custom overlays
The OverlayTrigger
component is great for most use cases, but as a higher level abstraction it can lack the flexibility needed to build more nuanced or custom behaviors into your Overlay components. For these cases it can be helpful to forgo the trigger and use the Overlay
component directly.
Use Overlay instead of Tooltip and Popover
You don't need to use the provided Tooltip
or Popover
components. Creating custom overlays is as easy as wrapping some markup in an Overlay
component.
Props
Name | Type | Default | Description |
---|---|---|---|
animation | boolean | elementType | Fade | Use animation |
onEnter | function | Callback fired before the Overlay transitions in | |
onEntered | function | Callback fired after the Overlay finishes transitioning in | |
onEntering | function | Callback fired as the Overlay begins to transition in | |
onExit | function | Callback fired right before the Overlay transitions out | |
onExited | function | Callback fired after the Overlay finishes transitioning out | |
onExiting | function | Callback fired as the Overlay begins to transition out | |
onHide | function | A callback invoked by the overlay when it wishes to be hidden. Required if
| |
placement | one of: 'top' , 'right' , 'bottom' , 'left' | 'right' | Sets the direction of the Overlay. |
rootClose | boolean | false | Specify whether the overlay should trigger onHide when the user clicks outside the overlay |
show | boolean | false | Set the visibility of the Overlay |
Navigation
React-Bootstrap offers a variety of responsive, accessible components for setting up navigation both across your website and within your pages.
Nav, NavItem Navs
Navs come in two styles, pills
and tabs
. Disable a tab by adding disabled
.
Dropdown
Add dropdowns using the NavDropdown
component.
Stacked
They can also be stacked
vertically.
Justified
They can be justified
to take the full width of their parent.
Props
Nav
Name | Type | Default | Description |
---|---|---|---|
activeHref | string | Marks the child NavItem with a matching | |
activeKey | any | Marks the NavItem with a matching | |
bsClass | string | 'nav' | Base CSS class and prefix for the component. Generally one should only change |
bsStyle | one of: "tabs" , "pills" | Component visual or contextual style variants. | |
justified | all | false | |
navbar | boolean | Apply styling an alignment for use in a Navbar. This prop will be set automatically when the Nav is used inside a Navbar. | |
onSelect | function | A callback fired when a NavItem is selected.
| |
pullLeft | boolean | false | Float the Nav to the left. When |
pullRight | boolean | false | Float the Nav to the right. When |
role | string | ARIA role for the Nav, in the context of a TabContainer, the default will be set to "tablist", but can be overridden by the Nav when set explicitly. When the role is set to "tablist" NavItem focus is managed according to the ARIA authoring practices for tabs: https://www.w3.org/TR/2013/WD-wai-aria-practices-20130307/#tabpanel | |
stacked | boolean | false | NavItems are be positioned vertically. |
NavItem
Name | Type | Default | Description |
---|---|---|---|
active | boolean | false | |
disabled | boolean | false | |
eventKey | any | ||
href | string | ||
onClick | function | ||
onSelect | function | ||
role | string |
Navbar Navbars
Navbars are responsive meta components that serve as navigation headers for your application or site.
They also support all the different Bootstrap classes as properties. Just camelCase the css class and remove navbar from it.
For example navbar-fixed-top
becomes the property fixedTop
. The different properties are fixedTop
, fixedBottom
, staticTop
, inverse
, and fluid
.
You can also align elements to the right by specifying the pullRight
prop on the Nav
, and other sub-components.
Navbar Basic Example
Additional Import Options
The Navbar Header, Toggle, Brand, and Collapse components are available as static properties on the <Navbar>
component but you can also import them directly from the /lib
directory like: require("react-bootstrap/lib/NavbarHeader")
.
Responsive Navbars
To have a mobile-friendly Navbar, add a Navbar.Toggle
to your Header and wrap your Nav
s in a Navbar.Collapse
component. The Navbar
will automatically make the toggle control the collapse.
Set the defaultExpanded
prop to make the Navbar start expanded. Set collapseOnSelect
to make the Navbar collapse automatically when the user selects an item. You can also finely control the collapsing behavior by using the expanded
and onToggle
props.
Forms
Use the Navbar.Form
convenience component to apply proper margins and alignment to form components.
Text and Non-nav links
Loose text and links can be wraped in the convenience components: Navbar.Link
and Navbar.Text
Props
Navbar
Name | Type | Default | Description |
---|---|---|---|
bsStyle | one of: "default" , "inverse" | 'default' | Component visual or contextual style variants. |
collapseOnSelect | boolean | false | Sets The onSelect callback should be used instead for more complex operations
that need to be executed after the |
componentClass | elementType | 'nav' | Set a custom element for this component. |
expanded | boolean | controlled by: onToggle , initial prop: defaultExpanded Explicitly set the visiblity of the navbar body | |
fixedBottom | boolean | false | Create a fixed navbar along the bottom of the screen, that scrolls with the page |
fixedTop | boolean | false | Create a fixed navbar along the top of the screen, that scrolls with the page |
fluid | boolean | false | Allow the Navbar to fluidly adjust to the page or container width, instead of at the predefined screen breakpoints |
inverse | boolean | false | An alternative dark visual style for the Navbar |
onSelect | function | A callback fired when a descendant of a child
For basic closing behavior after all Note: If you are manually closing the navbar using this | |
onToggle | function | controls expanded A callback fired when the | |
role | string | ||
staticTop | boolean | false | Create a full-width navbar that scrolls away with the page |
Navbar.Toggle
Name | Type | Default | Description |
---|---|---|---|
children | node | The toggle content, if left empty it will render the default toggle (seen above). | |
onClick | function |
Breadcrumb, Breadcrumb.Item Breadcrumbs
Breadcrumbs are used to indicate the current page's location. Add active
attribute to active Breadcrumb.Item
.
Do not set both active
and href
attributes. active
overrides href
and span
element is rendered instead of a
.
Breadcrumbs Example
Props
Breadcrumb
component itself doesn't have any specific public properties
Breadcrumb.Item
Name | Type | Default | Description |
---|---|---|---|
active | boolean | false | If set to true, renders |
href | string |
| |
target | string |
| |
title | node |
|
Tabs, Tab, TabContainer, TabContent, TabPane Togglable tabs
Add quick, dynamic tab functionality to transition through panes of local content.
Uncontrolled
Allow the component to control its own state.
Controlled
Pass down the active state on render via props.
No animation
Set the animation
prop to false
Tabs with Dropdown
Custom Tab Layout
For more complex layouts the flexible TabContainer
, TabContent
, andTabPane
components along with any style of Nav
allow you to quickly piece together your own Tabs component with additional markup needed.
Just create a set of NavItems each with an eventKey
corresponding to the eventKey of a TabPane
. Wrap the whole thing in a TabContainer
and you have fully functioning custom tabs component. Check out the below example making use of the grid system and pills.
Props
Tabs
Name | Type | Default | Description |
---|---|---|---|
activeKey | any | controlled by: onSelect , initial prop: defaultActiveKey Mark the Tab with a matching | |
animation | boolean | true | |
bsStyle | one of: 'tabs' , 'pills' | 'tabs' | Navigation style |
id | requiredForA11y | ||
mountOnEnter | boolean | false | Wait until the first "enter" transition to mount tabs (add them to the DOM) |
onSelect | function | controls activeKey Callback fired when a Tab is selected.
| |
unmountOnExit | boolean | false | Unmount tabs (remove it from the DOM) when it is no longer visible |
Tab
Name | Type | Default | Description |
---|---|---|---|
animation | boolean | elementType | Use animation when showing or hiding | |
aria-labelledby | string | ||
bsClass | string | 'tab-pane' | Base CSS class and prefix for the component. Generally one should only change |
disabled | boolean | ||
eventKey | any | Uniquely identify the | |
id | string | ||
mountOnEnter | boolean | Wait until the first "enter" transition to mount the tab (add it to the DOM) | |
onEnter | function | Transition onEnter callback when animation is not | |
onEntered | function | Transition onEntered callback when animation is not | |
onEntering | function | Transition onEntering callback when animation is not | |
onExit | function | Transition onExit callback when animation is not | |
onExited | function | Transition onExited callback when animation is not | |
onExiting | function | Transition onExiting callback when animation is not | |
tabClassName | string | tabClassName is used as className for the associated NavItem | |
title | node | ||
unmountOnExit | boolean | Unmount the tab (remove it from the DOM) when it is no longer visible |
TabContainer
Name | Type | Default | Description |
---|---|---|---|
activeKey | any | controlled by: onSelect , initial prop: defaultActiveKey The | |
generateChildId | function | (eventKey, type) => `${this.props.id}-${type}-${key}` | A function that takes an The |
id | custom | HTML id attribute, required if no | |
onSelect | function | controls activeKey A callback fired when a tab is selected. |
TabContent
Name | Type | Default | Description |
---|---|---|---|
animation | boolean | elementType | true | Sets a default animation strategy for all children |
bsClass | string | 'tab' | Base CSS class and prefix for the component. Generally one should only change |
componentClass | elementType | 'div' | You can use a custom element type for this component. |
mountOnEnter | boolean | false | Wait until the first "enter" transition to mount tabs (add them to the DOM) |
unmountOnExit | boolean | false | Unmount tabs (remove it from the DOM) when they are no longer visible |
TabPane
Name | Type | Default | Description |
---|---|---|---|
animation | boolean | elementType | Use animation when showing or hiding | |
aria-labelledby | string | ||
bsClass | string | 'tab-pane' | Base CSS class and prefix for the component. Generally one should only change |
eventKey | any | Uniquely identify the | |
id | string | ||
mountOnEnter | boolean | Wait until the first "enter" transition to mount the tab (add it to the DOM) | |
onEnter | function | Transition onEnter callback when animation is not | |
onEntered | function | Transition onEntered callback when animation is not | |
onEntering | function | Transition onEntering callback when animation is not | |
onExit | function | Transition onExit callback when animation is not | |
onExited | function | Transition onExited callback when animation is not | |
onExiting | function | Transition onExiting callback when animation is not | |
unmountOnExit | boolean | Unmount the tab (remove it from the DOM) when it is no longer visible |
Pagination Pagination
Provide pagination links for your site or app with the multi-page pagination component. Set items
to the number of pages. activePage
prop dictates which page is active
More options
such as first
, last
, previous
, next
, boundaryLinks
and ellipsis
.
Props
Name | Type | Default | Description |
---|---|---|---|
activePage | number | 1 | |
boundaryLinks | boolean | false | When |
bsClass | string | 'pagination' | Base CSS class and prefix for the component. Generally one should only change |
buttonComponentClass | elementType | You can use a custom element for the buttons | |
ellipsis | boolean | node | true | When |
first | boolean | node | false | When |
items | number | 1 | |
last | boolean | node | false | When |
maxButtons | number | 0 | |
next | boolean | node | false | When |
onSelect | function | ||
prev | boolean | node | false | When |
Pager, Pager.Item Pager
Quick previous and next links.
Centers by default
Aligned
Set the previous
or next
prop to true
, to align left or right.
Disabled
Set the disabled
prop to true
to disable the link.
Props
Pager
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'pager' | Base CSS class and prefix for the component. Generally one should only change |
onSelect | function |
Pager.Item
Page layout
The components in this section offer different ways to structure and present data on your page.
Grid, Row, Col, Clearfix Grid system
Basic Grid
<Col xs={12} md={8} />
<Col xs={6} md={4} />
<Col xs={6} md={4} />
<Col xs={6} md={4} />
<Col xs={6} xsOffset={6} />
<Col md={6} mdPush={6} />
<Col md={6} mdPull={6} />
Clearfix
Below, the columns won't clear correctly in viewport sm
(768px ≤ width < 992px).
<Col sm={6} md={3} />
Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Donec hendrerit tempor tellus. Donec pretium posuere tellus. Proin quam nisl, tincidunt et, mattis eget, convallis nec, purus. Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus. Nulla posuere.
<Col sm={6} md={3} />
Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Donec hendrerit tempor tellus. Donec pretium posuere tellus. Proin quam nisl, tincidunt et, mattis eget, convallis nec, purus.
<Col sm={6} md={3} />
Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Donec hendrerit tempor tellus. Donec pretium posuere tellus. Proin quam nisl, tincidunt et, mattis eget, convallis nec, purus. Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus. Nulla posuere.
<Col sm={6} md={3} />
Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Donec hendrerit tempor tellus.
Introduce Clearfix
, set to visible for the viewports with issue, so that columns clear correctly.
<Col sm={6} md={3} />
Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Donec hendrerit tempor tellus. Donec pretium posuere tellus. Proin quam nisl, tincidunt et, mattis eget, convallis nec, purus. Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus. Nulla posuere.
<Col sm={6} md={3} />
Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Donec hendrerit tempor tellus. Donec pretium posuere tellus. Proin quam nisl, tincidunt et, mattis eget, convallis nec, purus.
<Clearfix visibleSmBlock />
<Col sm={6} md={3} />
Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Donec hendrerit tempor tellus. Donec pretium posuere tellus. Proin quam nisl, tincidunt et, mattis eget, convallis nec, purus. Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus. Nulla posuere.
<Col sm={6} md={3} />
Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Donec hendrerit tempor tellus.
Props
Grid
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'container' | Base CSS class and prefix for the component. Generally one should only change |
componentClass | elementType | 'div' | You can use a custom element for this component |
fluid | boolean | false | Turn any fixed-width grid layout into a full-width layout by this property. Adds |
Row
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'row' | Base CSS class and prefix for the component. Generally one should only change |
componentClass | elementType | 'div' | You can use a custom element type for this component. |
Col
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'col' | Base CSS class and prefix for the component. Generally one should only change |
componentClass | elementType | 'div' | You can use a custom element type for this component. |
lg | number | The number of columns you wish to span for Large devices Desktops (≥1200px) class-prefix | |
lgHidden | boolean | Hide column on Large devices Desktops adds class | |
lgOffset | number | Move columns to the right for Large devices Desktops class-prefix | |
lgPull | number | Change the order of grid columns to the left for Large devices Desktops class-prefix | |
lgPush | number | Change the order of grid columns to the right for Large devices Desktops class-prefix | |
md | number | The number of columns you wish to span for Medium devices Desktops (≥992px) class-prefix | |
mdHidden | boolean | Hide column on Medium devices Desktops adds class | |
mdOffset | number | Move columns to the right for Medium devices Desktops class-prefix | |
mdPull | number | Change the order of grid columns to the left for Medium devices Desktops class-prefix | |
mdPush | number | Change the order of grid columns to the right for Medium devices Desktops class-prefix | |
sm | number | The number of columns you wish to span for Small devices Tablets (≥768px) class-prefix | |
smHidden | boolean | Hide column on Small devices Tablets adds class | |
smOffset | number | Move columns to the right for Small devices Tablets class-prefix | |
smPull | number | Change the order of grid columns to the left for Small devices Tablets class-prefix | |
smPush | number | Change the order of grid columns to the right for Small devices Tablets class-prefix | |
xs | number | The number of columns you wish to span for Extra small devices Phones (<768px) class-prefix | |
xsHidden | boolean | Hide column on Extra small devices Phones adds class | |
xsOffset | number | Move columns to the right for Extra small devices Phones class-prefix | |
xsPull | number | Change the order of grid columns to the left for Extra small devices Phones class-prefix | |
xsPush | number | Change the order of grid columns to the right for Extra small devices Phones class-prefix |
Clearfix
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'clearfix' | Base CSS class and prefix for the component. Generally one should only change |
componentClass | elementType | 'div' | You can use a custom element type for this component. |
visibleLgBlock | boolean | Apply clearfix on Large devices Desktops adds class | |
visibleMdBlock | boolean | Apply clearfix on Medium devices Desktops adds class | |
visibleSmBlock | boolean | Apply clearfix on Small devices Tablets adds class | |
visibleXsBlock | boolean | Apply clearfix on Extra small devices Phones adds class |
Jumbotron Jumbotron
A lightweight, flexible component that can optionally extend the entire viewport to showcase key content on your site.
Hello, world!
This is a simple hero unit, a simple jumbotron-style component for calling extra attention to featured content or information.
Props
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'jumbotron' | Base CSS class and prefix for the component. Generally one should only change |
componentClass | elementType | 'div' | You can use a custom element type for this component. |
PageHeader Page header
A simple shell for an h1
to appropriately space out and segment sections of content on a page. It can utilize the h1
’s default small
element, as well as most other components (with additional styles).
Example page header Subtext for header
Props
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'page-header' | Base CSS class and prefix for the component. Generally one should only change |
ListGroup, ListGroupItem List group
List groups are a flexible and powerful component for displaying not only simple lists of elements, but complex ones with custom content.
Centers by default
- Item 1
- Item 2
- ...
Linked
Set the href
or onClick
prop on ListGroupItem
, to create a linked or clickable element.
Styling by state
Set the active
or disabled
prop to true
to mark or disable the item.
Styling by color
Set the bsStyle
prop to style the item
- Success
- Info
- Warning
- Danger
With header
Set the header
prop to create a structured item, with a heading and a body area.
With custom component children
When using ListGroupItems directly, ListGroup looks at whether the items have href or onClick props to determine which DOM elements to emit. However, with custom item components as children to ListGroup
, set thecomponentClass
prop to specify which element ListGroup
should output.
- Custom Child 1
- Custom Child 2
- Custom Child 3
Props
ListGroup
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'list-group' | Base CSS class and prefix for the component. Generally one should only change |
componentClass | elementType | You can use a custom element type for this component. If not specified, it will be treated as |
ListGroupItem
Name | Type | Default | Description |
---|---|---|---|
active | any | ||
bsClass | string | 'list-group-item' | Base CSS class and prefix for the component. Generally one should only change |
bsStyle | one of: "success" , "warning" , "danger" , "info" | Component visual or contextual style variants. | |
disabled | any | ||
header | node | ||
href | string | ||
listItem | boolean | false | |
onClick | function | ||
type | string |
Table Tables
Use the striped
, bordered
, condensed
and hover
props to customise the table.
# | First Name | Last Name | Username |
---|---|---|---|
1 | Mark | Otto | @mdo |
2 | Jacob | Thornton | @fat |
3 | Larry the Bird |
Responsive
Add responsive
prop to make them scroll horizontally up to small devices (under 768px). When viewing on anything larger than 768px wide, you will not see any difference in these tables.
# | Table heading | Table heading | Table heading | Table heading | Table heading | Table heading |
---|---|---|---|---|---|---|
1 | Table cell | Table cell | Table cell | Table cell | Table cell | Table cell |
2 | Table cell | Table cell | Table cell | Table cell | Table cell | Table cell |
3 | Table cell | Table cell | Table cell | Table cell | Table cell | Table cell |
Props
Name | Type | Default | Description |
---|---|---|---|
bordered | boolean | false | |
bsClass | string | 'table' | Base CSS class and prefix for the component. Generally one should only change |
condensed | boolean | false | |
hover | boolean | false | |
responsive | boolean | false | |
striped | boolean | false |
Panel, PanelGroup, Accordion Panels
Basic example
By default, all the <Panel />
does is apply some basic border and padding to contain some content.
You can pass on any additional properties you need, e.g. a custom onClick
handler, as it is shown in the example code. They all will apply to the wrapper div
element.
Collapsible Panel
Panel with heading
Easily add a heading container to your panel with the header
prop.
Panel title
Panel with footer
Pass buttons or secondary text in the footer
prop. Note that panel footers do not inherit colors and borders when using contextual variations as they are not meant to be in the foreground.
Contextual alternatives
Like other components, easily make a panel more meaningful to a particular context by adding a bsStyle
prop.
Panel title
Panel title
Panel title
Panel title
Panel title
Panel title
With tables and list groups
Add the fill
prop to <Table />
or <ListGroup />
elements to make them fill the panel.
- Item 1
- Item 2
- …
Controlled PanelGroups
PanelGroup
s can be controlled by a parent component. The activeKey
prop dictates which panel is open.
Uncontrolled PanelGroups
PanelGroup
s can also be uncontrolled where they manage their own state. The defaultActiveKey
prop dictates which panel is open when initially.
Multiple Expandable Panels
PanelGroup
s can contain multiple expandable panels.
Accordions
<Accordion />
aliases <PanelGroup accordion />
.
Props
Panels
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'panel' | Base CSS class and prefix for the component. Generally one should only change |
bsStyle | one of: "success" , "warning" , "danger" , "info" , "default" , "primary" | 'default' | Component visual or contextual style variants. |
collapsible | boolean | ||
defaultExpanded | boolean | false | |
eventKey | any | ||
expanded | boolean | ||
footer | node | ||
header | node | ||
headerRole | string | ||
id | string | number | ||
onEnter | function | ||
onEntered | function | ||
onEntering | function | ||
onExit | function | ||
onExited | function | ||
onExiting | function | ||
onSelect | function | ||
panelRole | string |
PanelGroup, Accordion
Name | Type | Default | Description |
---|---|---|---|
accordion | boolean | false | |
activeKey | any | ||
bsClass | string | 'panel-group' | Base CSS class and prefix for the component. Generally one should only change |
defaultActiveKey | any | ||
onSelect | function | ||
role | string |
Well Wells
Use the well as a simple effect on an element to give it an inset effect.
Optional classes
Control padding and rounded corners with two optional modifier classes.
Props
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'well' | Base CSS class and prefix for the component. Generally one should only change |
bsSize | one of: "lg" , "large" , "sm" , "small" | Component size variations. |
FormGroup, FormControl, ControlLabel Forms
The <FormControl>
component renders a form control with Bootstrap styling. The <FormGroup>
component wraps a form control with proper spacing, along with support for a label, help text, and validation state. To ensure accessibility, set controlId
on <FormGroup>
, and use <ControlLabel>
for the label.
The <FormControl>
component directly renders the <input>
or other specified component. If you need to access the value of an uncontrolled <FormControl>
, attach a ref
to it as you would with an uncontrolled input, then call ReactDOM.findDOMNode(ref)
to get the DOM node. You can then interact with that node as you would with any other uncontrolled input.
If your application contains a large number of form groups, we recommend building a higher-level component encapsulating a complete field group that renders the label, the control, and any other necessary components. We don't provide this out-of-the-box, because the composition of those field groups is too specific to an individual application to admit a good one-size-fits-all solution.
Props
FormGroup
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'form-group' | Base CSS class and prefix for the component. Generally one should only change |
bsSize | one of: "lg" , "large" , "sm" , "small" | Component size variations. | |
controlId | string | Sets | |
validationState | one of: 'success' , 'warning' , 'error' , null |
FormControl
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'form-control' | Base CSS class and prefix for the component. Generally one should only change |
bsSize | one of: "sm" , "small" , "lg" , "large" | Component size variations. | |
componentClass | elementType | 'input' | You can use a custom element type for this component. |
id | string | Uses | |
inputRef | function | Attaches a ref to the
| |
type | string | Only relevant if |
ControlLabel
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'control-label' | Base CSS class and prefix for the component. Generally one should only change |
htmlFor | string | Uses | |
srOnly | boolean | false |
Checkbox, Radio, FormControl.Static, HelpBlock Supported controls
Examples of standard form controls supported in an example form layout, using a custom <FieldGroup>
component. Use <FormControl>
for <input>
, <textarea>
, and <select>
. Use <Checkbox>
and <Radio>
for checkboxes and radios respectively, optionally with inline
to render multiple on the same line. Use <FormControl.Static>
for static text.
Props
Checkbox
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'checkbox' | Base CSS class and prefix for the component. Generally one should only change |
disabled | boolean | false | |
inline | boolean | false | |
inputRef | function | Attaches a ref to the
| |
title | string | '' | |
validationState | one of: 'success' , 'warning' , 'error' , null | Only valid if |
Radio
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'radio' | Base CSS class and prefix for the component. Generally one should only change |
disabled | boolean | false | |
inline | boolean | false | |
inputRef | function | Attaches a ref to the
| |
title | string | '' | |
validationState | one of: 'success' , 'warning' , 'error' , null | Only valid if |
FormControl.Static
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'form-control-static' | Base CSS class and prefix for the component. Generally one should only change |
componentClass | elementType | 'p' | You can use a custom element type for this component. |
HelpBlock
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'help-block' | Base CSS class and prefix for the component. Generally one should only change |
Form Form layout
Inline forms
Use <Form inline>
instead of <form>
. JSX strips whitespace between lines, so you will need to manually add spaces. Additionally, Bootstrap assigns inline form controls width: auto
by default, so you may need to set custom widths.
Horizontal forms
Use <Form horizontal>
instead of <form>
, then use <Col>
s to align labels and controls. Do not use <Row>
here, as <FormGroup>
will already serve as a grid row in a horizontal form.
Props
(only needed for horizontal or inline forms) Form
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'form' | Base CSS class and prefix for the component. Generally one should only change |
componentClass | elementType | 'form' | You can use a custom element type for this component. |
horizontal | boolean | false | |
inline | boolean | false |
InputGroup, InputGroup.Addon, InputGroup.Button Input groups
Input add-ons
Wrap your form control in an <InputGroup>
, then use for normal add-ons and for button add-ons. Exotic configurations may require CSS on your side.
Input sizes
Use bsSize
on <FormGroup>
or <InputGroup>
to change the size of inputs. It also works with add-ons and most other options.
Props
InputGroup
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'input-group' | Base CSS class and prefix for the component. Generally one should only change |
bsSize | one of: "lg" , "large" , "sm" , "small" | Component size variations. |
InputGroup.Addon
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'input-group-addon' | Base CSS class and prefix for the component. Generally one should only change |
InputGroup.Button
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'input-group-btn' | Base CSS class and prefix for the component. Generally one should only change |
FormControl.Feedback Validation states
Set validationState
to one of 'success'
, 'warning'
or 'error'
to show validation state. Set validationState
to null
(or undefined
) to hide validation state. Add <FormControl.Feedback>
for a feedback icon when validation state is set.
Props
FormControl.Feedback
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'form-control-feedback' | Base CSS class and prefix for the component. Generally one should only change |
Media content
The React-Bootstrap media content components offer ways to present images and other media to your users in a responsive way, along with support for styling those components.
Image Images
Shape
Use the rounded
, circle
and thumbnail
props to customise the image.
Responsive
Use the responsive
to scale image nicely to the parent element.
Props
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'img' | Base CSS class and prefix for the component. Generally one should only change |
circle | boolean | false | Sets image shape as circle |
responsive | boolean | false | Sets image as responsive image |
rounded | boolean | false | Sets image shape as rounded |
thumbnail | boolean | false | Sets image shape as thumbnail |
Thumbnail Thumbnails
Thumbnails are designed to showcase linked images with minimal required markup. You can extend the grid component with thumbnails.
Anchor Thumbnail
Creates an anchor wrapping an image.
Divider Thumbnail
Creates a divider wrapping an image and other children elements.
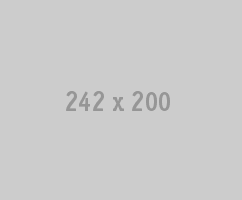
Thumbnail label
Description
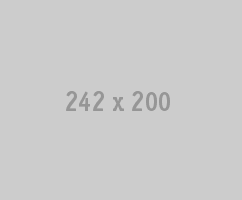
Thumbnail label
Description
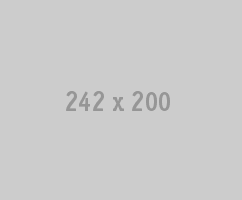
Thumbnail label
Description
Props
Name | Type | Default | Description |
---|---|---|---|
alt | string | alt property that is passed down to the image inside this component | |
bsClass | string | 'thumbnail' | Base CSS class and prefix for the component. Generally one should only change |
href | string | href property that is passed down to the image inside this component | |
onError | function | onError callback that is passed down to the image inside this component | |
onLoad | function | onLoad callback that is passed down to the image inside this component | |
src | string | src property that is passed down to the image inside this component |
ResponsiveEmbed Responsive embed
Allow browsers to determine video or slideshow dimensions based on the width of their containing block by creating an intrinsic ratio that will properly scale on any device.
You don't need to include frameborder="0"
in your iframe
s.
Either 16by9 or 4by3 aspect ratio via a16by9
or a4by3
attribute must be set.
Props
Name | Type | Default | Description |
---|---|---|---|
a16by9 | boolean | false | 16by9 aspect ratio |
a4by3 | boolean | false | 4by3 aspect ratio |
bsClass | string | 'embed-responsive' | Base CSS class and prefix for the component. Generally one should only change |
children required | element | This component requires a single child element |
Carousel, Carousel.Item, Carousel.Caption Carousels
Uncontrolled
Allow the component to control its own state.
Controlled
Pass down the active state on render via props.
Props
Carousel
Name | Type | Default | Description |
---|---|---|---|
activeIndex | number | ||
bsClass | string | 'carousel' | Base CSS class and prefix for the component. Generally one should only change |
controls | boolean | true | |
defaultActiveIndex | number | ||
direction | one of: 'prev' , 'next' | ||
indicators | boolean | true | |
interval | number | 5000 | The amount of time to delay between automatically cycling an item.
If |
nextIcon | node | <Glyphicon glyph="chevron-right" /> | |
nextLabel | string | 'Next' | Label shown to screen readers only, can be used to show the next element in the carousel. Set to null to deactivate. |
onSelect | function | Callback fired when the active item changes.
If this callback takes two or more arguments, the second argument will
be a persisted event object with | |
onSlideEnd | function | ||
pauseOnHover | boolean | true | |
prevIcon | node | <Glyphicon glyph="chevron-left" /> | |
prevLabel | string | 'Previous' | Label shown to screen readers only, can be used to show the previous element in the carousel. Set to null to deactivate. |
slide | boolean | true | |
wrap | boolean | true |
Carousel.Item
Name | Type | Default | Description |
---|---|---|---|
active | boolean | false | |
animateIn | boolean | false | |
animateOut | boolean | false | |
direction | one of: 'prev' , 'next' | ||
index | number | ||
onAnimateOutEnd | function |
Carousel.Caption
Media, Media.Left, Media.Right, Media.Heading, Media.List, Media.ListItem Media objects
Abstract object styles for building various types of components (like blog comments, Tweets, etc) that feature a left
or right
aligned image alongside textual content.
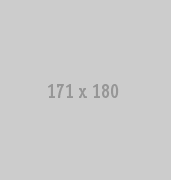
Media Heading
Cras sit amet nibh libero, in gravida nulla. Nulla vel metus scelerisque ante sollicitudin commodo. Cras purus odio, vestibulum in vulputate at, tempus viverra turpis. Fusce condimentum nunc ac nisi vulputate fringilla. Donec lacinia congue felis in faucibus.
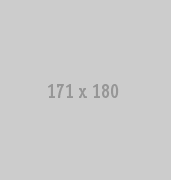
Media Heading
Cras sit amet nibh libero, in gravida nulla. Nulla vel metus scelerisque ante sollicitudin commodo. Cras purus odio, vestibulum in vulputate at, tempus viverra turpis. Fusce condimentum nunc ac nisi vulputate fringilla. Donec lacinia congue felis in faucibus.
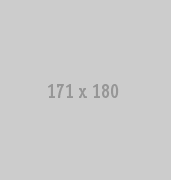
Nested Media Heading
Cras sit amet nibh libero, in gravida nulla. Nulla vel metus scelerisque ante sollicitudin commodo. Cras purus odio, vestibulum in vulputate at, tempus viverra turpis. Fusce condimentum nunc ac nisi vulputate fringilla. Donec lacinia congue felis in faucibus.
Media Heading
Cras sit amet nibh libero, in gravida nulla. Nulla vel metus scelerisque ante sollicitudin commodo. Cras purus odio, vestibulum in vulputate at, tempus viverra turpis. Fusce condimentum nunc ac nisi vulputate fringilla. Donec lacinia congue felis in faucibus.
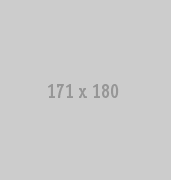
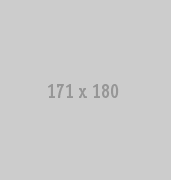
Media Heading
Cras sit amet nibh libero, in gravida nulla. Nulla vel metus scelerisque ante sollicitudin commodo. Cras purus odio, vestibulum in vulputate at, tempus viverra turpis. Fusce condimentum nunc ac nisi vulputate fringilla. Donec lacinia congue felis in faucibus.
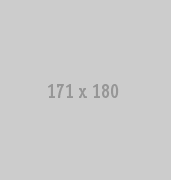
Media Alignment
The images or other media can be aligned top, middle, or bottom. The default is top aligned.
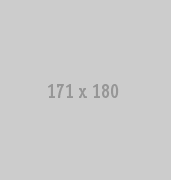
Top aligned media
Cras sit amet nibh libero, in gravida nulla. Nulla vel metus scelerisque ante sollicitudin commodo. Cras purus odio, vestibulum in vulputate at, tempus viverra turpis. Fusce condimentum nunc ac nisi vulputate fringilla. Donec lacinia congue felis in faucibus.
Donec sed odio dui. Nullam quis risus eget urna mollis ornare vel eu leo. Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus.
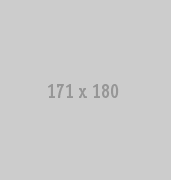
Middle aligned media
Cras sit amet nibh libero, in gravida nulla. Nulla vel metus scelerisque ante sollicitudin commodo. Cras purus odio, vestibulum in vulputate at, tempus viverra turpis. Fusce condimentum nunc ac nisi vulputate fringilla. Donec lacinia congue felis in faucibus.
Donec sed odio dui. Nullam quis risus eget urna mollis ornare vel eu leo. Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus.
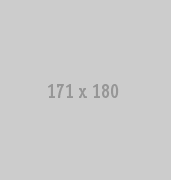
Bottom aligned media
Cras sit amet nibh libero, in gravida nulla. Nulla vel metus scelerisque ante sollicitudin commodo. Cras purus odio, vestibulum in vulputate at, tempus viverra turpis. Fusce condimentum nunc ac nisi vulputate fringilla. Donec lacinia congue felis in faucibus.
Donec sed odio dui. Nullam quis risus eget urna mollis ornare vel eu leo. Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus.
Media list
You can use media inside list (useful for comment threads or articles lists).
Media heading
Cras sit amet nibh libero, in gravida nulla. Nulla vel metus scelerisque ante sollicitudin commodo. Cras purus odio, vestibulum in vulputate at, tempus viverra turpis.
Nested media heading
Cras sit amet nibh libero, in gravida nulla. Nulla vel metus scelerisque ante sollicitudin commodo. Cras purus odio, vestibulum in vulputate at, tempus viverra turpis.
Nested media heading
Cras sit amet nibh libero, in gravida nulla. Nulla vel metus scelerisque ante sollicitudin commodo. Cras purus odio, vestibulum in vulputate at, tempus viverra turpis.
Nested media heading
Cras sit amet nibh libero, in gravida nulla. Nulla vel metus scelerisque ante sollicitudin commodo. Cras purus odio, vestibulum in vulputate at, tempus viverra turpis.
Props
Media
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'media' | Base CSS class and prefix for the component. Generally one should only change |
componentClass | elementType | 'div' | You can use a custom element type for this component. |
Media.Left
Media.Right
Media.Heading
Media.Body
Miscellaneous components
React-Bootstrap also offers various standalone components that can be used to present specific, relevant kinds of information across your pages.
Glyphicon Glyphicons
Use them in buttons, button groups for a toolbar, navigation, or prepended form inputs.
Props
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'glyphicon' | Base CSS class and prefix for the component. Generally one should only change |
glyph required | string | An icon name without "glyphicon-" prefix. See e.g. http://getbootstrap.com/components/#glyphicons |
Label Labels
Create a <Label>label</Label>
to highlight information
Label New
Label New
Label New
Label New
Label New
Label New
Available variations
Add any of the below mentioned modifier classes to change the appearance of a label.
Props
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'label' | Base CSS class and prefix for the component. Generally one should only change |
bsStyle | one of: "success" , "warning" , "danger" , "info" , "default" , "primary" | 'default' | Component visual or contextual style variants. |
Badge Badges
Easily highlight new or unread items by adding a <Badge>
to links, Bootstrap navs, and more.
Badges 42
Cross-browser compatibility
Unlike in regular Bootstrap, badges self collapse even in Internet Explorer 8.
Props
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'badge' | Base CSS class and prefix for the component. Generally one should only change |
pullRight | boolean | false |
Alert Alert messages
Basic alert styles.
Closeable alerts
just pass in a onDismiss
function.
Oh snap! You got an error!
Change this and that and try again. Duis mollis, est non commodo luctus, nisi erat porttitor ligula, eget lacinia odio sem nec elit. Cras mattis consectetur purus sit amet fermentum.
or
Props
Name | Type | Default | Description |
---|---|---|---|
bsClass | string | 'alert' | Base CSS class and prefix for the component. Generally one should only change |
bsStyle | one of: "success" , "warning" , "danger" , "info" | 'info' | Component visual or contextual style variants. |
closeLabel | string | 'Close alert' | |
onDismiss | function |
ProgressBar Progress bars
Provide up-to-date feedback on the progress of a workflow or action with simple yet flexible progress bars.
Basic example
Default progress bar.
With label
Add a label
prop to show a visible percentage. For low percentages, consider adding a min-width to ensure the label's text is fully visible.
Screenreader only label
Add a srOnly
prop to hide the label visually.
Contextual alternatives
Progress bars use some of the same button and alert classes for consistent styles.
Striped
Uses a gradient to create a striped effect. Not available in IE8.
Animated
Add active
prop to animate the stripes right to left. Not available in IE9 and below.
Stacked
Nest <ProgressBar />
s to stack them.
ProgressBar
Name | Type | Default | Description |
---|---|---|---|
active | boolean | false | |
bsClass | string | 'progress-bar' | Base CSS class and prefix for the component. Generally one should only change |
bsStyle | one of: "success" , "warning" , "danger" , "info" | Component visual or contextual style variants. | |
children | onlyProgressBar | ||
label | node | ||
max | number | 100 | |
min | number | 0 | |
now | number | ||
srOnly | boolean | false | |
striped | boolean | false |
Utilities
React-Bootstrap also exposes certain utility components used internally. They can be used to enhance your own custom components as well.
Collapse, Fade Transitions
Transition components animate their children transitioning in and out.
Collapse
Add a collapse toggle animation to an element or component.
Smoothing animations
If you're noticing choppy animations, and the component that's being collapsed has non-zero margin or padding, try wrapping the contents of your <Collapse>
inside a node with no margin or padding, like the <div>
in the example below. This will allow the height to be computed properly, so the animation can proceed smoothly.
Props
Name | Type | Default | Description |
---|---|---|---|
dimension | one of: 'height' , 'width' | function | 'height' | The dimension used when collapsing, or a function that returns the dimension Note: Bootstrap only partially supports 'width'!
You will need to supply your own CSS animation for the |
getDimensionValue | function | getDimensionValue | Function that returns the height or width of the animating DOM node Allows for providing some custom logic for how much the Collapse component should animate in its specified dimension. Called with the current dimension prop value and the DOM node. |
in | boolean | false | Show the component; triggers the expand or collapse animation |
mountOnEnter | boolean | false | Wait until the first "enter" transition to mount the component (add it to the DOM) |
onEnter | function | Callback fired before the component expands | |
onEntered | function | Callback fired after the component has expanded | |
onEntering | function | Callback fired after the component starts to expand | |
onExit | function | Callback fired before the component collapses | |
onExited | function | Callback fired after the component has collapsed | |
onExiting | function | Callback fired after the component starts to collapse | |
role | string | ARIA role of collapsible element | |
timeout | number | 300 | Duration of the collapse animation in milliseconds, to ensure that finishing callbacks are fired even if the original browser transition end events are canceled |
transitionAppear | boolean | false | Run the expand animation when the component mounts, if it is initially shown |
unmountOnExit | boolean | false | Unmount the component (remove it from the DOM) when it is collapsed |
Fade
Add a fade animation to a child element or component.
Props
Name | Type | Default | Description |
---|---|---|---|
in | boolean | false | Show the component; triggers the fade in or fade out animation |
mountOnEnter | boolean | false | Wait until the first "enter" transition to mount the component (add it to the DOM) |
onEnter | function | Callback fired before the component fades in | |
onEntered | function | Callback fired after the has component faded in | |
onEntering | function | Callback fired after the component starts to fade in | |
onExit | function | Callback fired before the component fades out | |
onExited | function | Callback fired after the component has faded out | |
onExiting | function | Callback fired after the component starts to fade out | |
timeout | number | 300 | Duration of the fade animation in milliseconds, to ensure that finishing callbacks are fired even if the original browser transition end events are canceled |
transitionAppear | boolean | false | Run the fade in animation when the component mounts, if it is initially shown |
unmountOnExit | boolean | false | Unmount the component (remove it from the DOM) when it is faded out |
Custom Styles
The bsStyle
prop, available in many components in React-Bootstrap, is used to map to a Bootstrap class for styling; for example, the Bootstrap class used for Button
is `btn-${bsStyle}`
. Use bootstrapUtils
to create a custom class that is used in lieu of the classes provided by Bootstrap:
Missing components
We've intentionally omitted a few components from React-Bootstrap. Don't worry, though – we cover what to do in this section.
Affix
Use <AutoAffix>
or <Affix>
from react-overlays.
There isn't really any additional Bootstrap markup associated with affixes, so we didn't add a Bootstrap-specific affix class. The upstream ones already give you everything you need.
Scrollspy
Setting up a scrollspy in idiomatic React requires wiring up a number of components across your entire page, both to handle elements scrolling in and to wire that up to the navigation. It's a poor fit for a component library, because it's not a standalone component.
To implement this functionality, use a library like React Waypoint along with a bit of your own state management. You can check out how we implemented it on the side panel here by reading the docs source.